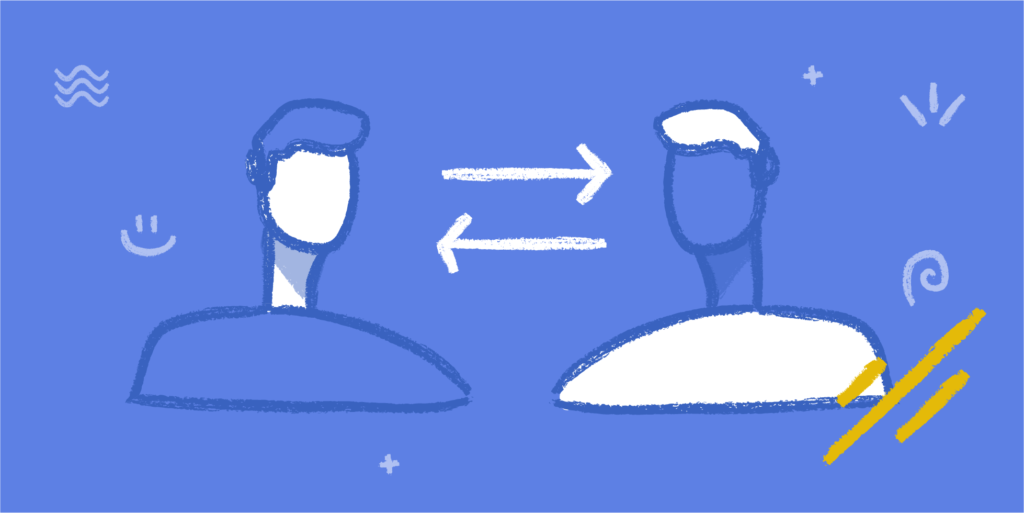
Symfony CRM – Let’s Add Customers
This is the second part of our custom CRM. Last article we learned how to set up the application. We will now start with our customer manager controller.
Here are the steps
- Create a new controller
-
php bin/console make:controller mainController
-
- Let’s create a basic menu on our application that will allow us to navigate the site
- A simple unordered list should suffice, I made one float to the left with the main content on the right side
- Add a link so that we can create a new customer, on your Customer Controller add the following code to add another route
-
/*** @Route(“/customer-manager/new-customer”, name=”newCustomer”)*/publicfunctionnewCustomer(){return$this->render(‘customer_manager/newCustomer.html.twig’, [‘controller_name’ => ‘CustomerManagerController’,]);}
- Make sure to add this before your index route for proper redirection
-
- Create a new TWIG file at
-
templates/customer_manager/newCustomer.html.twig
-
- Let’s start working now on connecting to our database, lets install the required files by running
-
composer require symfony/orm-pack
-
composer require –dev symfony/maker-bundle
-
- Set up your database connection information at your .env file
- Run the following command to set up your database
-
php bin/console doctrine:database:create
-
- Now lets create an entity on the database
-
php bin/console make:entity
-
- Now you have a file at
- src/Entity/Customer.php
- Run the following command to complete the database migration
-
php bin/console doctrine:migrations:migrate
-
- Now lets create a way to add customers to your database
- Start by creating a new route where your form will be submitted to
- Example: /customer-manager/add-customer
- Here is an example code to add a new customer to try the connection
-
use Doctrine\ORM\EntityManagerInterface;use Symfony\Component\HttpFoundation\Response;use App\Entity\Customer;
-
/*** @Route(“/customer-manager/add-customer”, name=”addCustomer”)*/public functioncreateProduct(): Response{// you can fetch the EntityManager via $this->getDoctrine()// or you can add an argument to the action: createProduct(EntityManagerInterface $entityManager)$entityManager = $this->getDoctrine()->getManager();$customer = newCustomer();$customer->setFullName(‘Glberto Cortez’);$customer->setEMail(‘save@test.com’);$customer->setPhone(‘(619) 339 9309’);$customer->setEid(‘2’);$objDateTime = newDateTime(‘NOW’);$customer->setDateCreated($objDateTime);$customer->setDateModified($objDateTime);// tell Doctrine you want to (eventually) save the Product (no queries yet)$entityManager->persist($customer);// actually executes the queries (i.e. the INSERT query)$entityManager->flush();returnnewResponse(‘Saved new product with id ‘.$customer->getId());}
-
- You should get the customer id back if it was successful
- Start by creating a new route where your form will be submitted to
- Now let’s create a new route so that we can retrieve specific customer information. Let’s try by retrieving the name of the last customer we added, here is the code:
-
/*** @Route(“/customer/{customerID}”, name=”showCustomer”)*/publicfunctionshow($customerID){$customer = $this->getDoctrine()->getRepository(Customer::class)->find($customerID);if (!$customer) {throw$this->createNotFoundException(‘No product found for id ‘.$customerID);}returnnewResponse(‘Check out this great customer ‘.$customer->getFullName());// or render a template// in the template, print things with {{ customer.name }}// return $this->render(‘customer/customer.html.twig’, [‘customer’ => $customer]);}
-
- Now just create a new form on your New Customer route that was previously created and submit it to the Add Customer route. Update the code to store the submitted information to:
-
use Symfony\Component\HttpFoundation\Request;
-
$request = Request::createFromGlobals();// you can fetch the EntityManager via $this->getDoctrine()// or you can add an argument to the action: createCustomer(EntityManagerInterface $entityManager)$entityManager = $this->getDoctrine()->getManager();$customer = newCustomer();$customer->setFullName( $request->get(‘customerFullName’) );$customer->setEMail( $request->get(‘customerPhone’) );$customer->setPhone( $request->get(‘customerEmail’) );$customer->setEid( $request->get(‘customerExternalId’) );$objDateTime = newDateTime(‘NOW’);$customer->setDateCreated($objDateTime);$customer->setDateModified($objDateTime);// tell Doctrine you want to (eventually) save the Product (no queries yet)$entityManager->persist($customer);// actually executes the queries (i.e. the INSERT query)$entityManager->flush();returnnewResponse(‘Saved new product with id ‘.$customer->getId());
-
- Now you are able to create new customers, add some CSS styling and you have the first piece of your customer relationship management application.
Let me know if this article helped you or if you have any questions. As well for any PHP, Symfony, MySQL JavaScript, React or other web project make sure to contact us so that we can help you with the development.