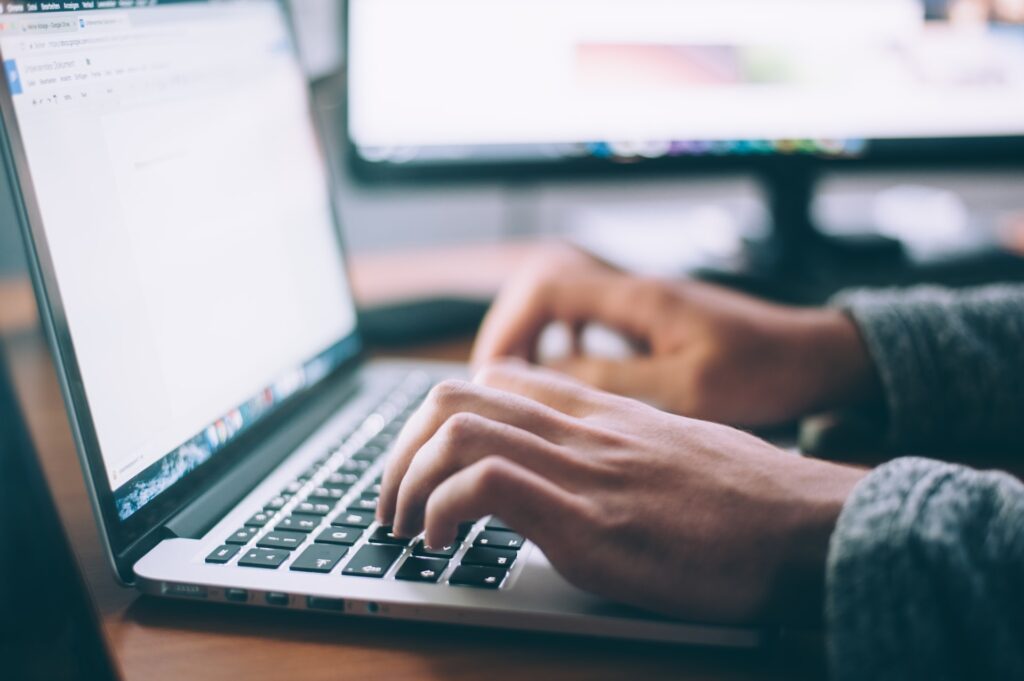
Quick Review: Creating a form in Symfony 5
Creating a form in Symfony 5 is a very simple process, just follow my instructions below. I will guide you in replacing the HTML form we used on our Symfony 4 CRM project.
If you have any questions please do let me know.
- Let’s make sure you have the required files on your project, run the following composer command
-
composer require symfony/form
-
- Now let’s use the built-in template system to create our form
-
php bin/console make:form
- Complete the requested information, I called it NewCustomer and bounded it to our previously created Customer model class.
-
- You can find the new form that was created at:
-
App\Form\NewCustomerType;
- Open the file and review it, it should have all of the model class properties
-
- Change your new customer route to the following:
-
$customer = new Customer();
$formNewCustomer = $this->createForm(NewCustomerType::class, $customer, [
‘action’ => $this->generateUrl(‘addCustomer’),’method’ => ‘POST’,
]);
return $this->render(‘customer_manager/newCustomer.html.twig’, [
‘form’ => $formNewCustomer->createView(),
]); - This will tell your controller to generate a new form using the NewCustomerType form class that was generated previously. We are as well telling the system that it is a POST form that will go to the ‘addCustomer’ route once submitted. Finally, we are creating the form view and passing it to the TWIG template file.
-
- Go to your twig template file and remove your old form, add the following command
-
{{ form(form) }}
- Just like that, refresh your new customer route page and make sure that the new form is showing.
-
- This is one of the most basic ways to add a Symfony Form to your application. Don’t forget it stills needs to be styled and most importantly, you need to code in a submit button at your NewCustomerType form class.
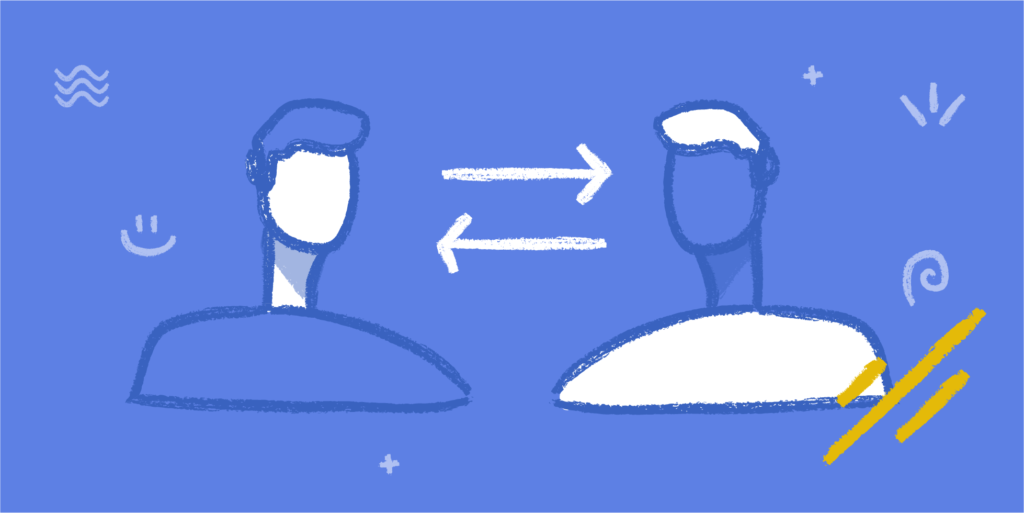
Symfony CRM – Let’s Add Customers
This is the second part of our custom CRM. Last article we learned how to set up the application. We will now start with our customer manager controller.
Here are the steps
- Create a new controller
-
php bin/console make:controller mainController
-
- Let’s create a basic menu on our application that will allow us to navigate the site
- A simple unordered list should suffice, I made one float to the left with the main content on the right side
- Add a link so that we can create a new customer, on your Customer Controller add the following code to add another route
-
/*** @Route(“/customer-manager/new-customer”, name=”newCustomer”)*/publicfunctionnewCustomer(){return$this->render(‘customer_manager/newCustomer.html.twig’, [‘controller_name’ => ‘CustomerManagerController’,]);}
- Make sure to add this before your index route for proper redirection
-
- Create a new TWIG file at
-
templates/customer_manager/newCustomer.html.twig
-
- Let’s start working now on connecting to our database, lets install the required files by running
-
composer require symfony/orm-pack
-
composer require –dev symfony/maker-bundle
-
- Set up your database connection information at your .env file
- Run the following command to set up your database
-
php bin/console doctrine:database:create
-
- Now lets create an entity on the database
-
php bin/console make:entity
-
- Now you have a file at
- src/Entity/Customer.php
- Run the following command to complete the database migration
-
php bin/console doctrine:migrations:migrate
-
- Now lets create a way to add customers to your database
- Start by creating a new route where your form will be submitted to
- Example: /customer-manager/add-customer
- Here is an example code to add a new customer to try the connection
-
use Doctrine\ORM\EntityManagerInterface;use Symfony\Component\HttpFoundation\Response;use App\Entity\Customer;
-
/*** @Route(“/customer-manager/add-customer”, name=”addCustomer”)*/public functioncreateProduct(): Response{// you can fetch the EntityManager via $this->getDoctrine()// or you can add an argument to the action: createProduct(EntityManagerInterface $entityManager)$entityManager = $this->getDoctrine()->getManager();$customer = newCustomer();$customer->setFullName(‘Glberto Cortez’);$customer->setEMail(‘save@test.com’);$customer->setPhone(‘(619) 339 9309’);$customer->setEid(‘2’);$objDateTime = newDateTime(‘NOW’);$customer->setDateCreated($objDateTime);$customer->setDateModified($objDateTime);// tell Doctrine you want to (eventually) save the Product (no queries yet)$entityManager->persist($customer);// actually executes the queries (i.e. the INSERT query)$entityManager->flush();returnnewResponse(‘Saved new product with id ‘.$customer->getId());}
-
- You should get the customer id back if it was successful
- Start by creating a new route where your form will be submitted to
- Now let’s create a new route so that we can retrieve specific customer information. Let’s try by retrieving the name of the last customer we added, here is the code:
-
/*** @Route(“/customer/{customerID}”, name=”showCustomer”)*/publicfunctionshow($customerID){$customer = $this->getDoctrine()->getRepository(Customer::class)->find($customerID);if (!$customer) {throw$this->createNotFoundException(‘No product found for id ‘.$customerID);}returnnewResponse(‘Check out this great customer ‘.$customer->getFullName());// or render a template// in the template, print things with {{ customer.name }}// return $this->render(‘customer/customer.html.twig’, [‘customer’ => $customer]);}
-
- Now just create a new form on your New Customer route that was previously created and submit it to the Add Customer route. Update the code to store the submitted information to:
-
use Symfony\Component\HttpFoundation\Request;
-
$request = Request::createFromGlobals();// you can fetch the EntityManager via $this->getDoctrine()// or you can add an argument to the action: createCustomer(EntityManagerInterface $entityManager)$entityManager = $this->getDoctrine()->getManager();$customer = newCustomer();$customer->setFullName( $request->get(‘customerFullName’) );$customer->setEMail( $request->get(‘customerPhone’) );$customer->setPhone( $request->get(‘customerEmail’) );$customer->setEid( $request->get(‘customerExternalId’) );$objDateTime = newDateTime(‘NOW’);$customer->setDateCreated($objDateTime);$customer->setDateModified($objDateTime);// tell Doctrine you want to (eventually) save the Product (no queries yet)$entityManager->persist($customer);// actually executes the queries (i.e. the INSERT query)$entityManager->flush();returnnewResponse(‘Saved new product with id ‘.$customer->getId());
-
- Now you are able to create new customers, add some CSS styling and you have the first piece of your customer relationship management application.
Let me know if this article helped you or if you have any questions. As well for any PHP, Symfony, MySQL JavaScript, React or other web project make sure to contact us so that we can help you with the development.
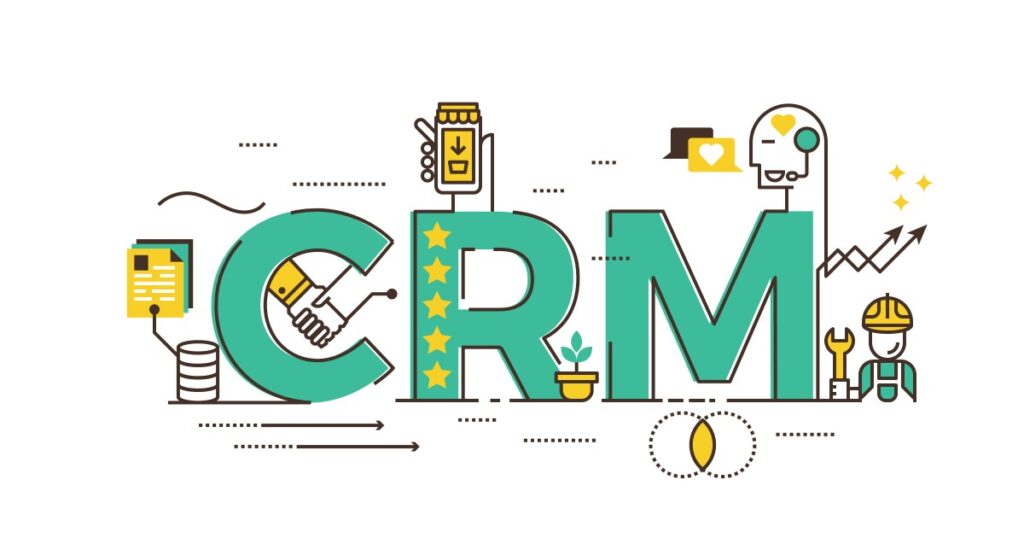
Starting a custom CRM with Symfony 5
These are some of my notes on how to create a custom client-relationship manager (CRM) with Symfony5. I hope that this assists you in making a better project, let me know if you know of any better way to achieve this.
This is the steps to follow, which do not only work just for this type of application but for most full-size web applications built with Symfony 5:
- Download and install Symfony5 from https://symfony.com/download
- Start a new project with the following command
-
symfony new crm –full
- Where crm is the project name
-
- Make sure it loads properly by starting the development server
-
symfony serve -d
- If the command it’s successful it will provide you the HTTPS URL where the server is loaded onto
-
- Create a new default controller for the application
-
php bin/console make:controller mainController
- You can use the following command to obtain a list of things that you can easily do via you CLI
- php bin/console list
- You can use the following command to obtain a list of things that you can easily do via you CLI
-
- Open your new controller using your editor of preference to edit the new controller, in this, you will see the default code. The location will be
-
src/Controller/MainController
-
- Start by changing the route URL to “/” and refreshing your development server. It should now show the new home page from the MainController
- Let’s install WebPack into our application by running
-
composer require webpack-encore
-
yarn install
-
- Let’s execute WebPack by running
-
yarn watch
- It is very important you install webpack-encore before any other yarn dependency. Failure to do so will result in an error and in a problem when running the command
-
- After the build it’s complete, we can then add what will be our JavaScript and CSS main files it onto our main template file
- We do this by opening our base TWIG template file saved at
-
templates/base.html.twig
-
- Then we add the following
- Into the stylesheets, block add
-
{{ encore_entry_link_tags(‘app’) }}
-
- Into the JavaScript, block add
-
{{ encore_entry_script_tags(‘app’) }}
-
- Into the stylesheets, block add
- Once added refresh your app, you should see now a Gray background. If you check your console you should see a log with a message “Hello Webpack Encore!”
- We do this by opening our base TWIG template file saved at
- Now let’s install Bootstrap so that we can give your application a better shell to be displayed in
-
yarn add bootstrap –dev
-
- After it is installed, we need to add the following line into the top our CSS main file located at “assets/css/app.css”
-
@import “~bootstrap”;
- If you refresh your application, you will notice the change in font size and style
-
This is how I would properly set up initially a Symfony5 CRM application. I hope it helps you, more lists are to follow to assist you in building your project.