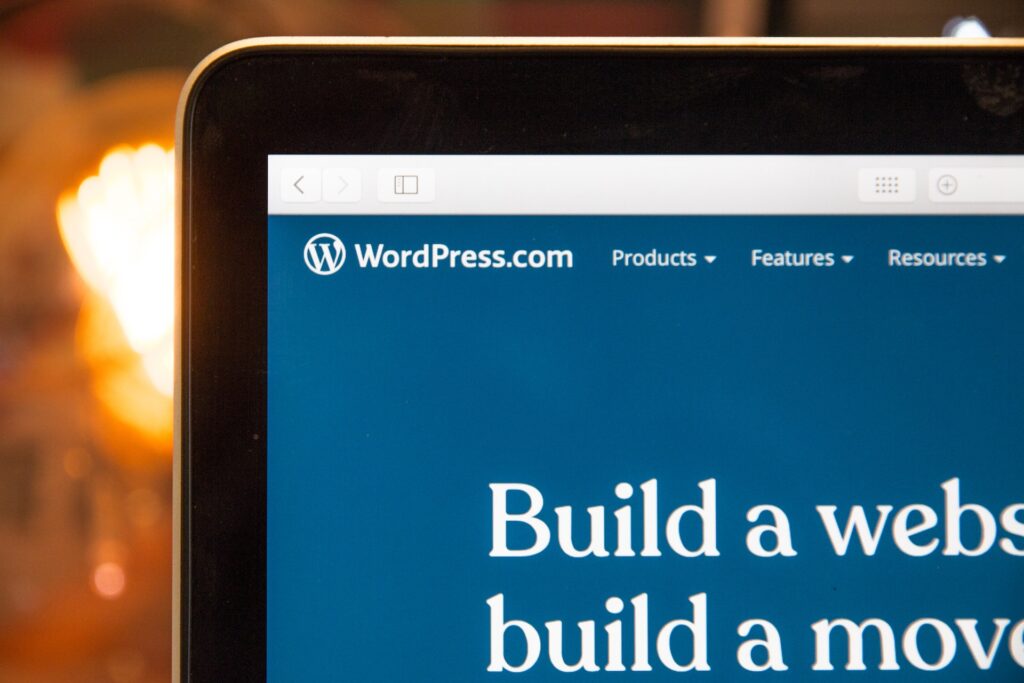
WordPress Functions.php 101
There are many things that can be added to the functions.php file of a WordPress theme, but some of the most common and useful include:
- Theme support: You can add support for various features like post thumbnails, custom headers, and menus, by using add_theme_support() function.
- Enqueueing scripts and styles: You can use the wp_enqueue_script() and wp_enqueue_style() functions to add CSS and JavaScript files to your theme.
- Registering and enqueueing custom post types and taxonomies: You can use the register_post_type() and register_taxonomy() functions to create custom post types and taxonomies, and then use the wp_enqueue_script() and wp_enqueue_style() functions to add the necessary CSS and JavaScript files.
- Customizing the WordPress login page: You can use the login_enqueue_scripts hook to add custom CSS and JavaScript files to the login page.
- Customizing the WordPress dashboard: You can use the wp_dashboard_setup hook to customize the WordPress dashboard by adding custom widgets, removing default widgets, and more.
- Adding custom widgets: You can use the register_sidebar() and register_widget() functions to create custom widgets to be used in sidebars and footers of your theme.
- Customizing the excerpt: You can use the excerpt_length and excerpt_more filters to customize the appearance of post excerpts on your site.
- Customizing the pagination: You can use the paginate_links filter to customize the appearance of pagination links.
These are just a few examples of the many things that can be added to the functions.php file of a WordPress theme. The possibilities are endless, and the best things to add will depend on the specific needs of your theme.
Theme Support
In WordPress, theme support refers to the ability to enable certain features within a theme that are provided by the core WordPress software. These features are often related to the presentation and layout of a website, and can include things like post thumbnails, custom headers, and custom menus.
When a theme is activated, it can call the add_theme_support() function in its functions.php file to register support for various features. This tells WordPress that the theme is designed to work with these features, and that they should be enabled when the theme is active.
For example, a theme might call add_theme_support( ‘post-thumbnails’ ) to register support for post thumbnails. This would allow the theme to use featured images for posts and pages. Another example is calling add_theme_support( ‘menus’ ) to register support for custom menus, so that the theme can use the WordPress menu management system.
By calling add_theme_support() for different features, a theme can ensure that all the necessary functionality is available for use. Theme developers can check whether a certain feature is supported by using the current_theme_supports() function.
It’s worth noting that some features are automatically enabled by WordPress when a theme is activated, and do not require a call to add_theme_support() to work.
The add_theme_support() function can be used to register support for a variety of features in WordPress. Some examples include:
- Post Thumbnails: Allows the theme to use featured images for posts and pages. Example: add_theme_support( ‘post-thumbnails’ );
- Custom Headers: Allows the theme to use custom headers for the site. Example: add_theme_support( ‘custom-header’ );
- Custom Backgrounds: Allows the theme to use custom backgrounds for the site. Example: add_theme_support( ‘custom-background’ );
- Custom Logos: Allows the theme to use custom logos for the site. Example: add_theme_support( ‘custom-logo’ );
- Title Tag: Allows WordPress to handle the site title tag. Example: add_theme_support( ‘title-tag’ );
- Automatic Feed Links: Allows the theme to add automatic feed links to the <head> section of the site. Example: add_theme_support( ‘automatic-feed-links’ );
- Post Formats: Allows the theme to use post formats for different types of content. Example: add_theme_support( ‘post-formats’, array( ‘aside’, ‘gallery’, ‘video’ ) );
- HTML5: Allows the theme to use HTML5 markup for certain areas of the site. Example: add_theme_support( ‘html5’, array( ‘search-form’, ‘comment-form’, ‘comment-list’ ) );
- Customizer: Allows the theme to use the WordPress Customizer for theme options. Example: add_theme_support( ‘customize-selective-refresh-widgets’ );
- Widgets: Allows the theme to use widgets. Example: add_theme_support( ‘widgets’ );
- Nav Menus: Allows the theme to use custom navigation menus. Example: add_theme_support( ‘menus’ );
- Block Styles: Allows the theme to style blocks in the Gutenberg editor. Example: add_theme_support( ‘wp-block-styles’ );
- Alignments: Allows the theme to control the alignments of elements in the Gutenberg editor. Example: add_theme_support( ‘align-wide’ );
To allow for featured images in WordPress posts, you can use the add_theme_support() function in your theme’s functions.php file.
add_theme_support( ‘post-thumbnails’ );
This code adds support for featured images in posts, allowing you to set a featured image for each post in the post editor.
It’s worth noting that this function only enables featured images in posts, if you want to enable featured images in other post types such as pages, you’ll need to use the set_post_thumbnail_size() function to set the dimensions of the featured images and use the add_image_size() function to create custom image sizes that can be used for different areas of your theme.
set_post_thumbnail_size( 50, 50 ); add_image_size( ‘featured-image’, 600, 400, true );
This code sets the default featured image size to 50×50 pixels and creates a custom image size called ‘featured-image’ with dimensions of 600×400 pixels and it crops the image to maintain the aspect ratio.
It’s also worth noting that if your theme is using an older version of WordPress and doesn’t have support for featured images, you will have to add this feature by using a plugin that adds support for featured images in posts.
These are just a few examples of the many features that can be enabled using the add_theme_support() function, depending on the version of WordPress and the theme. The best things to add will depend on the specific needs of your theme.
Enqueuing scripts and styles
Enqueuing scripts and styles in WordPress refers to the process of adding CSS and JavaScript files to a WordPress website. These files are typically added to the <head> section of the website’s HTML code and are used to control the layout, design, and functionality of the site.
To enqueue scripts and styles in a WordPress theme, you can use the wp_enqueue_script() and wp_enqueue_style() functions, respectively. These functions are typically added to the functions.php file of the theme, and are executed by the wp_enqueue_scripts action hook.
Here is an example of how to use the wp_enqueue_style() function to add a stylesheet to a WordPress theme:
function my_theme_styles() { wp_enqueue_style( ‘my-theme-style’, get_stylesheet_uri() ); } add_action( ‘wp_enqueue_scripts’, ‘my_theme_styles’ );
This code first defines a function called my_theme_styles() that uses the wp_enqueue_style() function to enqueue a stylesheet called my-theme-style using the get_stylesheet_uri() function. This function retrieves the URI of the current theme’s stylesheet.
Then, the add_action() function is used to add the my_theme_styles() function to the wp_enqueue_scripts action hook. This hook is triggered by WordPress when it is loading the scripts and styles for the website, and it will execute the function, adding the stylesheet to the website.
Similarly, you can use the wp_enqueue_script() function to add a JavaScript file to your theme. Here is an example of how to use the function:
function my_theme_scripts() { wp_enqueue_script( ‘my-theme-script’, get_template_directory_uri() . ‘/js/my-theme-script.js’, array( ‘jquery’ ) ); } add_action( ‘wp_enqueue_scripts’, ‘my_theme_scripts’ );
This code first defines a function called my_theme_scripts() that uses the wp_enqueue_script() function to enqueue a JavaScript file called my-theme-script.js located in the /js folder of the theme directory. The last parameter of the function is an array of dependencies that the script needs to function, in this case is jquery library.
Then, the add_action() function is used to add the my_theme_scripts() function to the wp_enqueue_scripts action hook, this will execute the function, adding the JavaScript file to the website.
It’s worth noting that by using these functions, WordPress will handle the dependency management, versioning and location of the files for you. Additionally, you can also use the wp_register_style() and wp_register_script() functions before wp_enqueue_style() and wp_enqueue_script() respectively to register your files and then enqueue them where you need them, this will make sure that your files are properly added to the head of the document and will be loaded in every page of your WordPress site.
Registering and enqueueing custom post types and taxonomies
In WordPress, custom post types and taxonomies are used to create new types of content and organize existing content in ways that are specific to a particular website or application. Custom post types are used to create new types of posts, such as portfolios, events, or team members. Taxonomies, on the other hand, are used to create new ways of grouping and categorizing content, such as product categories or location tags.
To register a custom post type or taxonomy, you can use the register_post_type() and register_taxonomy() functions, respectively. These functions are typically added to the functions.php file of the theme.
Here is an example of how to use the register_post_type() function to register a custom post type called “Books”:
function my_custom_post_type() { $args = array( ‘label’ => ‘Books’, ‘public’ => true, ‘show_ui’ => true, ‘capability_type’ => ‘post’, ‘hierarchical’ => false, ‘rewrite’ => array(‘slug’ => ‘books’), ‘query_var’ => true, ‘menu_icon’ => ‘dashicons-book’, ‘supports’ => array( ‘title’, ‘editor’, ‘excerpt’, ‘trackbacks’, ‘custom-fields’, ‘comments’, ‘revisions’, ‘thumbnail’, ‘author’, ‘page-attributes’, ) ); register_post_type( ‘books’, $args ); } add_action( ‘init’, ‘my_custom_post_type’ );
This code first defines a function called my_custom_post_type() that uses the register_post_type() function to register a custom post type called “Books”. The function takes an array of arguments that define the properties of the custom post type, such as its label, whether it’s public or not, whether it should be shown in the WordPress admin interface, and which capabilities it should have. Additionally, it sets the ‘rewrite’ parameter to set the URL structure for the custom post type, ‘query_var’ to make the custom post type available for querying, ‘menu_icon’ to set a custom icon for the post type in the admin menu and ‘supports’ to specify which features of the post editor should be supported.
Then, the add_action() function is used to add the my_custom_post_type() function to the init action hook, which is triggered by WordPress when it is initializing the theme. This will execute the function and register the custom post type.
Similarly, you can use the register_taxonomy() function to register a custom taxonomy. Here is an example of how to use the function to register a custom taxonomy called “Genres” for the custom post type “Books”
function my_custom_taxonomy() { $args = array( ‘label’ => ‘Genres’, ‘public’ => true, ‘show_ui’ => true, ‘hierarchical’ => true, ‘rewrite’ => array( ‘slug’ => ‘genre’ ), ); register_taxonomy( ‘genre’, ‘books’, $args ); }
add_action( ‘init’, ‘my_custom_taxonomy’ );
This code is similar to the custom post type example, it first defines a function called my_custom_taxonomy() that uses the register_taxonomy() function to register a custom taxonomy called “Genres”. The function takes an array of arguments that define the properties of the custom taxonomy, such as its label, whether it’s public or not, whether it should be shown in the WordPress admin interface, and whether it should be hierarchical or not. Additionally, it sets the ‘rewrite’ parameter to set the URL structure for the custom taxonomy.
Then, the add_action() function is used to add the my_custom_taxonomy() function to the init action hook, which is triggered by WordPress when it is initializing the theme. This will execute the function and register the custom taxonomy.
Once you have registered the custom post type and taxonomy, you can use them to create new posts and terms and you can also use the wp_enqueue_script() and wp_enqueue_style() functions to add CSS and JavaScript files that are specific to the custom post type and taxonomy to your theme, this will help you to style and add functionality to your custom post type and taxonomy pages.
Customizing the WordPress login page
Customizing the WordPress login page refers to the process of modifying the appearance and functionality of the login page in a WordPress website. The login page is the page where users enter their username and password to access the WordPress dashboard.
To customize the WordPress login page, you can use the login_enqueue_scripts action hook, which is triggered by WordPress when the login page is loaded. This hook allows you to add custom CSS and JavaScript files to the login page.
Here is an example of how to use the login_enqueue_scripts action hook to add a custom stylesheet to the login page:
function my_custom_login_styles() { wp_enqueue_style( ‘my-login-style’, get_template_directory_uri() . ‘/css/my-login-style.css’ ); } add_action( ‘login_enqueue_scripts’, ‘my_custom_login_styles’ );
This code first defines a function called my_custom_login_styles() that uses the wp_enqueue_style() function to enqueue a stylesheet called my-login-style located in the /css folder of the theme directory.
Then, the add_action() function is used to add the my_custom_login_styles() function to the login_enqueue_scripts action hook, this will execute the function, adding the stylesheet to the login page.
You can also use the login_enqueue_scripts action hook to add custom JavaScript to the login page. Here is an example of how to use the hook to add a custom script:
function my_custom_login_scripts() { wp_enqueue_script( ‘my-login-script’, get_template_directory_uri() . ‘/js/my-login-script.js’ ); } add_action( ‘login_enqueue_scripts’, ‘my_custom_login_scripts’ );
This code first defines a function called my_custom_login_scripts() that uses the wp_enqueue_script() function to enqueue a JavaScript file called my-login-script
located in the /js folder of the theme directory.
Then, the add_action() function is used to add the my_custom_login_scripts() function to the login_enqueue_scripts action hook, this will execute the function, adding the JavaScript file to the login page.
In addition to customizing the look and feel of the login page using CSS and JavaScript, you can also customize the functionality of the login page by using the login_form and authenticate filters. These filters allow you to modify the HTML of the login form, change the behavior of the login process, and add custom validation logic.
Here is an example of how to use the login_form filter to add a custom field to the login form:
function my_custom_login_field( $content ) { $content .= ‘<p> <label for=”my_custom_field”>My Custom Field</label> <input type=”text” name=”my_custom_field” id=”my_custom_field” class=”input” value=”” size=”20″ /> </p>’; return $content; } add_filter( ‘login_form’, ‘my_custom_login_field’ );
This code first defines a function called my_custom_login_field() that takes a parameter called $content, which is the HTML of the login form. The function uses the .= operator to append a new field to the form. It has a label, an input type and its name, id, class and its value and size.
Then, the add_filter() function is used to add the my_custom_login_field() function to the login_form filter, this will execute the function and add the custom field to the login form.
It’s worth noting that when customizing the login page, it’s important to be careful with the changes you make, as it could break the login process or make it vulnerable to security threats. Additionally, if you are going to customize the functionality of the login page, make sure to test it thoroughly and keep a backup of your website in case something goes wrong.
Another thing to consider is that if you are using a caching plugin, it’s possible that the changes you made to the login page won’t be visible right away, as the cache might need to be cleared.
In summary, customizing the WordPress login page can be done by using the login_enqueue_scripts action hook to add custom CSS and JavaScript files, and by using filters such as login_form and authenticate to modify the HTML of the login form and change the behavior of the login process. However, make sure to test the changes you made, and be mindful of security concerns, and also consider caching.
Another thing you can do to customize the WordPress login page is to change the logo on the login page. By default, the logo on the login page is the WordPress logo, but you can change it to your own logo. You can do this by using the login_headerurl and login_headertitle filters.
Here’s an example of how to use these filters to change the logo on the login page:
function my_custom_login_logo() { return get_bloginfo( ‘url’ ); } add_filter( ‘login_headerurl’, ‘my_custom_login_logo’ ); function my_custom_login_logo_url_title() { return ‘My Custom Title’; } add_filter( ‘login_headertitle’, ‘my_custom_login_logo_url_title’ );
This code first defines a function called my_custom_login_logo() that returns the URL of the website using the get_bloginfo() function. Then, the add_filter() function is used to add the my_custom_login_logo() function to the login_headerurl filter, this will execute the function and change the logo link on the login page to the website URL.
Second function defined is called my_custom_login_logo_url_title() that returns a custom title for the logo, Then, the add_filter() function is used to add the my_custom_login_logo_url_title() function to the login_headertitle filter, this will execute the function and change the title for the logo link to the custom title.
In addition to that you can also change the logo by simply replacing the default logo in the theme’s images folder, you can use CSS to hide the default logo and display your custom logo instead.
It’s worth noting that you can also use a plugin that allows you to customize the login page. These plugins can be a good option if you’re not comfortable editing code, or if you want a more extensive set of customization options.
Customizing the WordPress dashboard
Customizing the WordPress dashboard refers to the process of modifying the appearance and functionality of the WordPress admin area. The dashboard is the first screen that users see when they log in to the WordPress backend, it contains widgets that provide an overview of the site’s content and statistics, as well as links to other areas of the admin area.
There are several ways to customize the WordPress dashboard, some of the most common methods include:
- Removing or rearranging the default widgets: You can use the wp_dashboard_setup action hook to remove or rearrange the default widgets that appear on the dashboard. This can be useful if you want to simplify the dashboard or remove widgets that are not relevant to your site.
- Adding custom widgets: You can use the wp_add_dashboard_widget function to add custom widgets to the dashboard. This can be useful if you want to add functionality or display information that is not available in the default widgets.
- Customizing the layout: You can use CSS to change the appearance of the dashboard, such as colors, fonts, and spacing. This can be useful if you want to match the look and feel of the dashboard to your site’s branding.
- Modifying the menu: You can use the admin_menu action hook to add, remove or rearrange the items in the admin menu. This can be useful if you want to hide certain sections of the admin area, or create custom sections.
Here is an example of how to use the wp_add_dashboard_widget function to add a custom widget to the dashboard:
function my_custom_dashboard_widget() { wp_add_dashboard_widget( ‘my_custom_dashboard_widget’, ‘My Custom Widget’, ‘my _custom_dashboard_widget_content’ ); } add_action( ‘wp_dashboard_setup’, ‘my_custom_dashboard_widget’ );
function my_custom_dashboard_widget_content() { echo “Hello, this is my custom dashboard widget!”; }
This code first defines a function called `my_custom_dashboard_widget` that uses the `wp_add_dashboard_widget` function to add a custom widget to the dashboard. The function takes three parameters: – The first parameter is a unique ID for the widget – The second parameter is the title of the widget – The third parameter is the function that will be used to display the content of the widget. Then, the `add_action()` function is used to add the `my_custom_dashboard_widget` function to the `wp_dashboard_setup` action hook, which is triggered by WordPress when the dashboard is loaded. This will execute the function and add the custom widget to the dashboard. The second function `my_custom_dashboard_widget_content()` is used to define the content that will be displayed inside the custom widget, in this case, it just prints a simple text message. It’s worth noting that when customizing the dashboard, it’s important to be careful with the changes you make, as it could break the functionality of the dashboard or make it difficult for users to navigate. Additionally, if you are going to customize the functionality of the dashboard, make sure to test it thoroughly and keep a backup of your website in case something goes wrong.
Adding custom widgets
Adding custom widgets to the WordPress dashboard is a great way to display additional information or functionality that is specific to your website or application. To add a custom widget, you can use the wp_add_dashboard_widget function, which takes three parameters:
- The first parameter is a unique ID for the widget. This ID is used to identify the widget and should be unique to prevent conflicts with other widgets.
- The second parameter is the title of the widget. This is the text that will be displayed in the header of the widget.
- The third parameter is the function that will be used to display the content of the widget. This function should print or echo the HTML or content that you want to display in the widget.
Here is an example of how to use the wp_add_dashboard_widget function to add a custom widget that displays the number of comments on the website:
function my_custom_dashboard_widget() { wp_add_dashboard_widget( ‘my_custom_dashboard_widget’, ‘Comment Count’, ‘my_custom_dashboard_widget_content’ ); } add_action( ‘wp_dashboard_setup’, ‘my_custom_dashboard_widget’ ); function my_custom_dashboard_widget_content() { $comments_count = wp_count_comments(); echo “Total comments: ” . $comments_count->approved; }
This code first defines a function called my_custom_dashboard_widget that uses the wp_add_dashboard_widget function to add a custom widget to the dashboard. The function takes three parameters:
- The first parameter is a unique ID for the widget, in this case, “my_custom_dashboard_widget”
- The second parameter is the title of the widget, in this case, “Comment Count”
- The third parameter is the function that will be used to display the content of the widget, in this case, “my_custom_dashboard_widget_content”
Then, the add_action() function is used to add the my_custom_dashboard_widget function to the wp_dashboard_setup action hook, which is triggered by WordPress when the dashboard is loaded. This will execute the function and add the custom widget to the dashboard.
The second function my_custom_dashboard_widget_content() is used to define the content that will be displayed inside the custom widget. In this case, it uses the wp_count_comments() function to get the total number of comments on the website and then it prints the number of approved comments on the website.
You can also use PHP and HTML inside the content function to add more complex and interactive widgets, such as forms, tables, and charts.
It’s worth noting that you can also use a plugin that allows you to add custom widgets to the dashboard. These plugins can be a good option if you’re not comfortable editing code, or if you want a more extensive set of customization options.
Customizing the excerpt
The excerpt is a summary of a post’s content, it’s usually used in places where the full content of a post would be too long, such as in archive pages, search results, and RSS feeds. In WordPress, the excerpt is generated automatically by taking the first few sentences of a post’s content, but you can also define a custom excerpt for each post.
There are several ways to customize the excerpt in WordPress, some of the most common methods include:
- Defining a custom excerpt: You can define a custom excerpt for each post by using the Excerpt field in the post editor, this field allows you to write a summary of the post’s content that will be used as the excerpt.
- Modifying the default length of the excerpt: By default, WordPress uses the first 55 words of a post’s content as the excerpt, but you can change this by using the excerpt_length filter. This filter allows you to define the number of words that should be used as the excerpt.
- Modifying the default read more text: By default, WordPress adds a “Read more” link to the end of the excerpt, but you can change this text by using the excerpt_more filter. This filter allows you to define the text that should be used as the read more link.
Here is an example of how to use the excerpt_length filter to change the length of the excerpt:
function my_custom_excerpt_length( $length ) { return 30; } add_filter( ‘excerpt_length’, ‘my_custom_excerpt_length’, 999 );
This code first defines a function called my_custom_excerpt_length that takes a parameter called $length, which is the default length of the excerpt. The function returns 30 which is the number of words that should be used as the excerpt.
Then, the add_filter() function is used to add the `my _custom_excerpt_lengthfunction to theexcerpt_length` filter, this will execute the function and change the default length of the excerpt to 30 words. The third parameter ‘999’ is the priority of the filter.
Here is an example of how to use the excerpt_more filter to change the read more text:
function my_custom_excerpt_more( $more ) { return ‘… <a href=”‘ . get_permalink() . ‘”>Read More</a>’; } add_filter( ‘excerpt_more’, ‘my_custom_excerpt_more’ );
This code first defines a function called my_custom_excerpt_more that takes a parameter called $more, which is the default read more text. The function returns the custom read more text which is ‘…Read More’ along with the link to the post.
Then, the add_filter() function is used to add the my_custom_excerpt_more function to the excerpt_more filter, this will execute the function and change the default read more text to the custom read more text.
It’s worth noting that these filters only affect the automatic excerpt, if you want to customize the excerpt for all the posts, you should do it manually or you can also use a plugin which can help you customize the excerpt for all the posts.
Customizing the pagination
Customizing the pagination in WordPress refers to the process of modifying the appearance and functionality of the navigation links that are used to split content into multiple pages. In WordPress, the pagination is generated automatically by the wp_link_pages() function, but you can customize it by modifying the arguments passed to this function or by using a custom pagination function.
Here is an example of how to use the wp_link_pages() function with custom arguments to modify the appearance of the pagination links:
wp_link_pages( array( ‘before’ => ‘<p>’ . __( ‘Pages:’ ), ‘after’ => ‘</p>’, ‘link_before’ => ”, ‘link_after’ => ”, ‘next_or_number’ => ‘number’, ‘separator’ => ‘ ‘, ‘nextpagelink’ => __( ‘Next page’ ), ‘previouspagelink’ => __( ‘Previous page’ ), ‘pagelink’ => ‘%’, ‘echo’ => 1 ) );
This code uses the wp_link_pages() function to output the pagination links. It passes an array of arguments to the function which modify the appearance of the links.
- ‘before’ and ‘after’ arguments are used to wrap the pagination links with a specific HTML element.
- ‘link_before’ and ‘link_after’ arguments are used to add a specific HTML element before and after each link.
- ‘next_or_number’ argument is used to display the links as numbers or next/previous links.
- ‘separator’ argument is used to separate the links by a specific character or HTML element.
- ‘nextpagelink’ and ‘previouspagelink’ arguments are used to change the text of the next and previous links.
- ‘pagelink’ argument is used to specify the format of the page number links.
- ‘echo’ argument is used to specify whether the function should print the links or return them as a string.
In addition to using the wp_link_pages() function with custom arguments, you can also use a custom pagination function to completely replace the default pagination. This method requires you to write a custom function that generates the HTML for the pagination links and then call that function in the template where you want the pagination to appear.
Here is an example of how to use a custom pagination function to generate the pagination links:
function my_custom_pagination() { global $wp_query; $total_pages = $wp_query->max_num_pages; if ($total_pages > 1){ $current_page = max(1, get_query_var(‘paged’)); echo ‘<div class=”pagination”>’; for ($i=1; $i <= $total_pages; $i++){ if ($i == $current_page) { echo ‘<span class=”current-page”>’.$i.'</span>’; } else { echo ‘<a href=”‘.get_pagenum_link($i).'”>’.$i.'</a>’; } } echo ‘</div>’; } }
This code first defines a function called my_custom_pagination that generates the HTML for the pagination links. It starts by getting the total number of pages and the current page number. Then it loops through all the pages and generates the HTML for each page. If the current page is equal to the current page of the loop, it prints the page number inside a <span> with the class ‘current-page’ otherwise it prints a link to the page inside an <a> tag.
You can then call this function in the template file where you want the pagination to appear. For example, you can call the function in the index.php file or in the archive.php file if you want the pagination to appear on the archive pages.
<?php my_custom_pagination(); ?>
It’s worth noting that when customizing the pagination, you should make sure that your custom function is compatible with the current theme and it’s responsive. Additionally, you should also test your custom pagination function with different types of content and with different numbers of pages to ensure that it’s working correctly and that it’s easy for users to navigate.