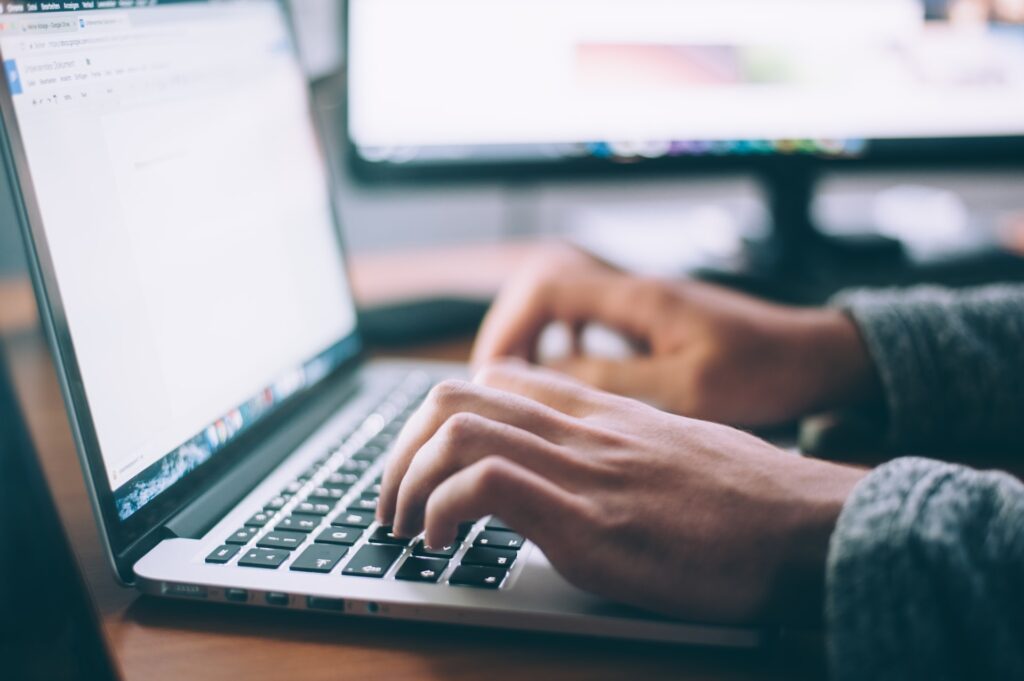
Quick Review: Creating a form in Symfony 5
Creating a form in Symfony 5 is a very simple process, just follow my instructions below. I will guide you in replacing the HTML form we used on our Symfony 4 CRM project.
If you have any questions please do let me know.
- Let’s make sure you have the required files on your project, run the following composer command
-
composer require symfony/form
-
- Now let’s use the built-in template system to create our form
-
php bin/console make:form
- Complete the requested information, I called it NewCustomer and bounded it to our previously created Customer model class.
-
- You can find the new form that was created at:
-
App\Form\NewCustomerType;
- Open the file and review it, it should have all of the model class properties
-
- Change your new customer route to the following:
-
$customer = new Customer();
$formNewCustomer = $this->createForm(NewCustomerType::class, $customer, [
‘action’ => $this->generateUrl(‘addCustomer’),’method’ => ‘POST’,
]);
return $this->render(‘customer_manager/newCustomer.html.twig’, [
‘form’ => $formNewCustomer->createView(),
]); - This will tell your controller to generate a new form using the NewCustomerType form class that was generated previously. We are as well telling the system that it is a POST form that will go to the ‘addCustomer’ route once submitted. Finally, we are creating the form view and passing it to the TWIG template file.
-
- Go to your twig template file and remove your old form, add the following command
-
{{ form(form) }}
- Just like that, refresh your new customer route page and make sure that the new form is showing.
-
- This is one of the most basic ways to add a Symfony Form to your application. Don’t forget it stills needs to be styled and most importantly, you need to code in a submit button at your NewCustomerType form class.